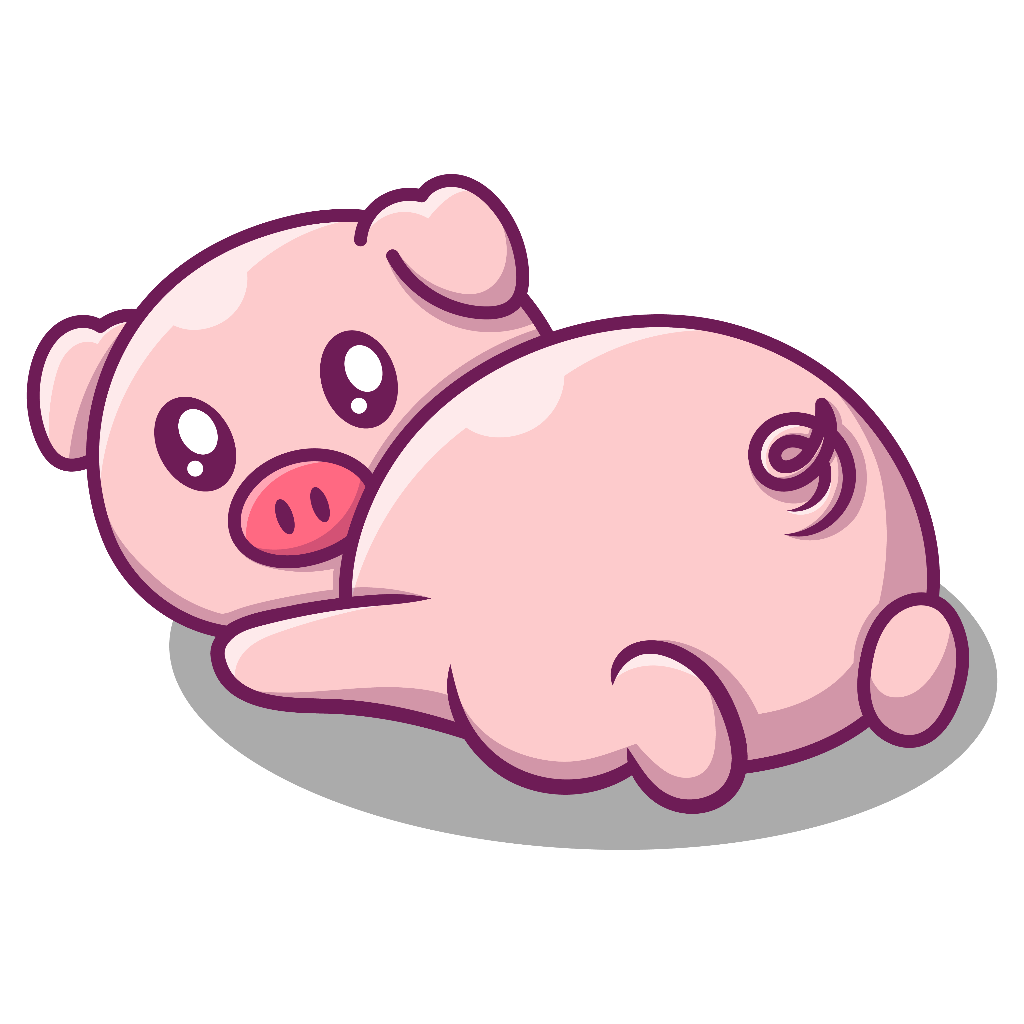
DigitalBacon (0.2.1)
This API document is meant for software developers using the DigitalBacon JavaScript module. Looking for the Application Guide?
For past versions of this document, please checkout the version you need from GitHub
Introduction
DigitalBacon is a library to create and deploy three.js scenes that support PC, Mobile, and VR sessions without all the boilerplate required to do it yourself. Additionally this library hopes to enable developers to create more complex scenes faster by exposing the renderer, scene, camera, helper functions, and more
How to Use
You can download the DigitalBacon module from GitHub under the build folder or using a package manager like npm. When importing it into your project, Digital Bacon requires three.js, three-mesh-ui, and three-mesh-bvh to be included in the import map. Please see below for an example that has the dependencies installed locally via npm
<script type="importmap">
{
"imports": {
"DigitalBacon": "/node_modules/digitalbacon/build/DigitalBacon.js",
"three": "/node_modules/three/build/three.module.js",
"three-mesh-ui": "/node_modules/three-mesh-ui/build/three-mesh-ui.module.js",
"three-mesh-bvh": "/node_modules/three-mesh-bvh/build/index.module.js"
}
}
</script>
Then in the script you want to use the module in, you can import either the attributes and functions you need individually or everything as so
import * as DigitalBacon from 'DigitalBacon';
DigitalBacon Module
Attributes
version |
String The version of DigitalBacon this module is |
Assets |
Object See Assets for more info |
AudioHandler |
Object See AudioHandler for more info |
EditorHelpers |
Object See EditorHelpers for more info |
InputHandler |
Object See InputHandler for more info |
Interactables |
Object See Interactables for more info |
LibraryHandler |
Object See LibraryHandler for more info |
MenuInputs |
Object See MenuInputs for more info |
PartyHandler |
Object See PartyHandler for more info |
ProjectHandler |
Object See ProjectHandler for more info |
PubSub |
Object See PubSub for more info |
SettingsHandler |
Object See SettingsHandler for more info |
UserController |
Object See UserController for more info |
Functions
setup(containerId, setupParams)
Sets up project without user editing enabled
Returns Promise<App> that resolves after initial assets have loaded
containerId |
String id of the div element you want DigitalBacon to render in |
setupParams |
Map Optional Click here for a detailed list of key/value pairs that can be provided |
setupEditor(containerId, projectFilePath)
Sets up project with user editing enabled
Returns Promise<App> that resolves after initial assets have loaded
containerId |
String id of the div element you want DigitalBacon to render in |
setupParams |
Map Optional Click here for a detailed list of key/value pairs that can be provided |
setupParams
projectFilePath |
String Optional File path of the Digital Bacon project you want to load on startup. If not provided, a scene with only ambient lighting will be created |
authUrl |
String Optional The authorization url provided to you by your party service to support multi-user experiences |
socketUrl |
String Optional The party url provided to you by your party service to support multi-user experiences |
onStart |
Function Optional Will be called upon the user clicking the start button |
getCamera()
Should only be run after Promise from setup() or setupEditor() resolves
Returns the Camera used for viewing the scene (See Three.js Camera Documentation for more details)
getDeviceType()
Should only be run after Promise from setup() or setupEditor() resolves
Returns one of "XR", "MOBILE", or "POINTER"
disableImmersion()
Disables immersive capabilities such as the UserController and Menu. Must be run before calling either setup() or setupEditor()
App
Functions
getRenderer()
Returns the Renderer (See Three.js WebGLRenderer Documentation for more details)
getScene()
Returns the Scene (See Three.js Scene Documentation for more details)
Assets
Click on the below links to see the source code for that asset
Attributes
- Asset
- AssetEntity
- AudioAsset
- CustomAsset
- CustomAssetEntity
- Component
- ImageAsset
- ModelAsset
- System
- VideoAsset
- Material
- BasicMaterial
- StandardMaterial
- ToonMaterial
- Texture
- BasicTexture
- CubeTexture
- Light
- AmbientLight
- DirectionalLight
- HemisphereLight
- PointLight
- SpotLight
- Shape
- BoxShape
- CircleShape
- ConeShape
- CylinderShape
- PlaneShape
- RingShape
- SphereShape
- TorusShape
AudioHandler
getListener()
Useful when needing to create a Custom Asset that uses audio
Returns the AudioListener that represents the virtual listener of all audio
setListenerParent(listenerParent)
Makes the AudioListener a child of the parent
listenerParent |
Object3D The three.js Object3D you want to set as the parent of the AudioListener |
EditorHelpers
Inherit from the provided EditorHelpers when creating your own assets to enable configuring your custom assets in the editor
Attributes
- AssetEntityHelper
- ComponentHelper
- CustomAssetHelper
- CustomAssetEntityHelper
- EditorHelper
- EditorHelperFactory
- MaterialHelper
- LightHelper
- ShapeHelper
- SystemHelper
- TextureHelper
InputHandler
addExtraControlsButton(id, name)
Adds html button to the bottom right of the screen
Returns an html button with the provided id and name
id |
String The id of the button you're creating |
name |
String The text on the button that will be seen by the user |
getExtraControlsButton(id)
Returns the html button with the provided id
id |
String The id of the button you've already created |
hideExtraControlsButton(id)
Sets the css display property of the html button to none
id |
String The id of the button you've already created |
showExtraControlsButton(id)
Sets the css display property of the html button to inline-block
id |
String The id of the button you've already created |
getXRGamepad(handedness)
Returns the XR Gamepad associated with the controller with the provided handedness if it exists
handedness |
String (LEFT or RIGHT) The handedness associated with the controller you want the XR Gamepad for |
isPointerPressed()
Returns a boolean that signifies if the mouse is currently being pressed down
isScreenTouched()
Returns a boolean that signifies if the touch screen is currently being touched
isKeyCodePressed(code)
Returns whether the key associated with the provided code is currently pressed on the keyboard
code |
String The code that represents a physical key on the keyboard. Please see here for more information |
Interactables
Make assets interactive by creating Interactables for them and adding them to their corresponding InteractableHandler
Attributes
- Interactable
- GripInteractable
- PointerInteractable
- GripInteractableHandler
- PointerInteractableHandler
LibraryHandler
loadAsset(filepath, callback)
Adds image/model to library
Returns Promise<assetId> that resolves after initial assets have loaded
filepath |
String File path of the image/model you want to add |
callback |
function Optional Callback that when called, will pass in the library asset id |
getType(assetId)
Returns the type of the corresponding asset. Will be one of "MODEL", "IMAGE", "LIGHT", "SHAPE", "MATERIAL", "TEXTURE", "COMPONENT", or "SYSTEM"
assetId |
String id of the asset in question |
MenuInputs
Object containing different Input Types. Particularly useful when defining new EditorHelpers for your custom assets. The tables below list the parameters you can provide for each type when defined for an EditorHelper. To see the actual parameters these Classes accept, please see the source code here
Attributes for use with EditorHelper
Required with MenuInputs for newly defined parameters
parameter |
String parameter name of the asset that this Checkbox affects |
name |
String User friendly name for this parameter. Optional when listing an inherited parameter |
AudioInput
No additional fields
CheckboxInput
suppressMenuFocusEvent |
Boolean Optional Suppresses the Menu Focus Event from being published |
ColorInput
CubeImageInput
No additional fields
EnumInput
map |
Object<String, Any> Map of user friendly names to their corresponding value to be used when setting the Asset's parameter |
EulerInput
ImageInput
MaterialInput
No additional fields
NumberInput
min |
Number Optional Maximum value this field can be set to |
max |
Number Optional Minimum value this field can be set to |
TextureInput
filter |
TextureType Optional Only allow user to select from textures of this type |
TextInput
Vector2Input
Vector3Input
No additional fields
PartyHandler
Functions
isHost()
Returns whether or not the user is the Party Host
addMessageHandler(id, handler, skipQueue)
Registers a callback function that triggers when messages to the provided id are received from peers
id |
String Id that is used to filter messages |
handler |
function(peer, message) Callback function triggered when messages with the provided id are found |
skipQueue |
Boolean Optional If true, will process message immediately upon receiving it instead of in the order all messages are being received |
addBufferMessageHandler(id, handler, skipQueue)
Same as addMessageHandler, but for ArrayBuffer messages
id |
8-bit Number | uuid String Id that is used to filter messages |
handler |
function(peer, message) Callback function triggered when messages with the provided id are found |
skipQueue |
Boolean Optional If true, will process message immediately upon receiving it instead of in the order all messages are being received |
publishFromFunction(publishFunction)
Typically used when you want to block other messages in the publish queue from being sent while an asynchronous process generates the message(s) you want to publish. See the following code snippet for an example
publishFunction |
function() Callback function triggered from the publish queue |
publishMessage(id, message, skipQueue, ...peerList)
Publishes a message to all or optionally some list of peers using the provided id
id |
String The id that is used to filter messages by message handlers |
message |
Object | String | Number The message being sent. If message is an object, the object must be parseable by JSON.parse() |
skipQueue |
Boolean Optional If true, will send message immediately |
...peerList |
Expanded List<Peers> Optional List of peers that you want to send the message to. If not provided, message will be sent to all peers |
publishBufferMessage(id, message, skipQueue, ...peerList)
Publishes an ArrayBuffer message to all or optionally some list of peers using the provided id
id |
8-bit Number | 128-bit uuid Uint8Array | uuid String The id that is used to filter messages by message handlers |
message |
ArrayBuffer The message being sent |
skipQueue |
Boolean Optional If true, will send message immediately |
...peerList |
Expanded List<Peers> Optional List of peers that you want to send the message to. If not provided, message will be sent to all peers |
addMessageHandlerLock()
Adds a lock to prevent messages from being processed by the message queue
Returns the lock that was created
removeMessageHandlerLock(lock)
Removes the lock from preventing messages being processed by the message queue
lock |
String The lock that was provided to you by addMessageHandlerLock() |
ProjectHandler
getAsset(id)
Returns a reference to the asset instance with the following id if it exists within the project
getSessionAsset(id)
Same as getAsset(id), except includes asset instances that have been deleted from the project as well
id |
String ID of the asset instance you want a reference to |
addNewAsset(assetId, params, ignorePublish, ignoreUndoRedo)
Adds a new instance to the project based on the assetId provided
Returns the asset instance that was created
assetId |
String ID of the asset you want to create that corresponds to some existing asset in the Library |
params |
object Optional Parameters used for creating the Asset |
ignorePublish |
Boolean Optional Whether the creation of this instance should not be broadcasted to other users. Defaults to false (will be broadcasted to other users) |
ignoreUndoRedo |
Boolean Optional Whether the creation of this instance should not be registered as an action that can be undone + redone. Defaults to false (can be undone + redone) |
addAsset(asset, ignorePublish, ignoreUndoRedo)
Adds the asset to the project
asset |
Asset Instance The asset you want to add to the project |
ignorePublish |
Boolean Optional Whether the addition of this instance to the project should not be broadcasted to other users. Defaults to false (will be broadcasted to other users) |
ignoreUndoRedo |
Boolean Optional Whether the addition of this instance to the project should not be registered as an action that can be undone + redone. Defaults to false (can be undone + redone) |
deleteAsset(asset, ignorePublish, ignoreUndoRedo)
Deletes the asset from the project
asset |
Asset Instance The asset you want to delete from the project |
ignorePublish |
Boolean Optional Whether the deletion of this instance should not be broadcasted to other users. Defaults to false (will be broadcasted to other users) |
ignoreUndoRedo |
Boolean Optional Whether the deletion of this instance should not be registered as an action that can be undone + redone. Defaults to false (can be undone + redone) |
load(filepath, successCallback, errorCallback)
Loads the provided Digital Bacon project
filepath |
String File path of the Digital Bacon project you want to load |
successCallback |
function Optional Callback function that is triggered after successfully loading the project file |
errorCallback |
function Optional Callback function that is triggered if an error occerred while loading the project file |
PubSub
Functions
subscribe(ownerId, topic, callback)
Registers a callback function that triggers when internal messages to the provided topic are received. A given owner can only have 1 subscription per topic
ownerId |
String An id used to identify the publisher/subscriber. If the same ownerId was used to publish to this topic, then this callback function won't be triggered |
topic |
String Topic that is listened to |
handler |
function(peer, message) Callback function triggered when messages with the provided topic are found |
unsubscribe(ownerId, topic)
Unregisters the callback function that was provided when subscribing with this same ownerId and topic
ownerId |
String The id that was used when subscribing to this same topic before |
topic |
String Topic that is listened to |
publish(ownerId, topic, message, urgent)
Publishes a message to the given topic
ownerId |
String An id used to identify the publisher/subscriber. If the same ownerId is subscribed to this topic, then that callback function won't be triggered |
topic |
String Topic that is published to |
message |
Any Data that you want to publish |
urgent |
Boolean Optional If you want the message to be published immediately, instead of during the publishing period that occurs once per frame |
SettingsHandler
Functions
setUserSetting(key, value, ignorePublish)
Updates the value of a user setting
key |
String They key associated with the user setting such as "Enable Flying" |
value |
Any The value of the setting you want set to |
ignorePublish |
Boolean Optional If true, will suppress the "SETTINGS_UPDATED" message published through PubSub |
UserController
Functions
getAvatar()
Gets the Avatar of the user
getController(handedness)
Returns the XRController of user for the corresponding side. Returns null if the user is not on an XR device
handedness |
String (RIGHT or LEFT) Which XRController you want |
getHand(handedness)
Returns the XRHand of user for the corresponding side. Returns null if the user is not on an XR device
handedness |
String (RIGHT or LEFT) Which XRHand you want |